Advanced Routing Techniques in ASP.NET Core MVC
Updated on
May 10, 2024
Learn advanced ASP.NET Core MVC routing techniques for cleaner code and easier maintenance.
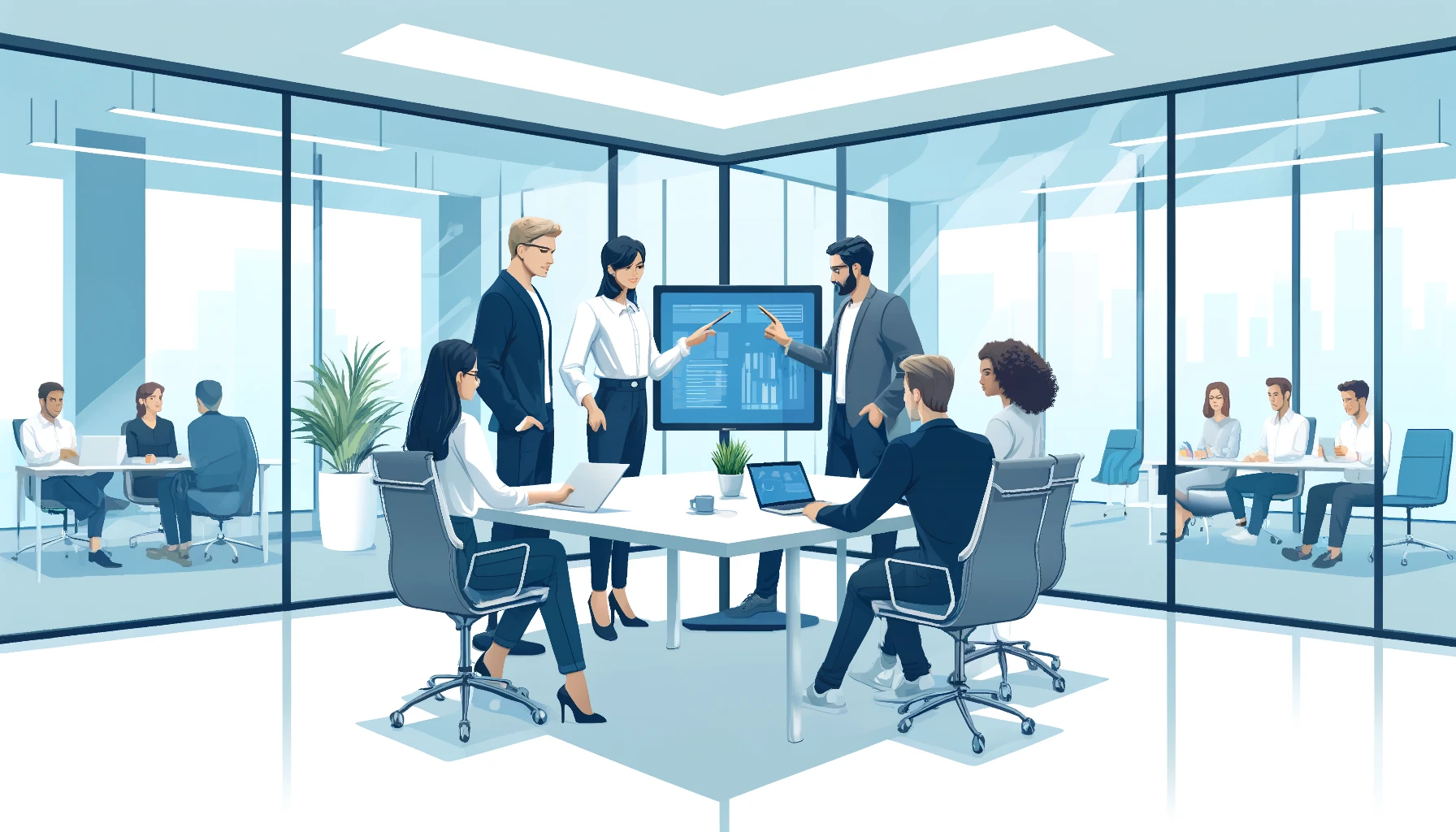
This tutorial provides an introduction to advanced routing techniques in ASP.NET Core MVC, focusing on the use of attribute routing. By implementing advanced routing, developers can better organize code and simplify the maintenance of applications. Let’s dive into the core concepts and practical implementations.
1. Understanding Routing in ASP.NET Core MVC
Routing in ASP.NET Core MVC is the process that decides which action method in a controller gets executed when a client makes a request. The default routing technique is conventional routing, but advanced scenarios often require more control, which is where attribute routing comes into play.
2. Basics of Attribute Routing
Attribute routing uses attributes to define routes directly on controllers and actions. This provides more granular control over the routes and can make them more readable and maintainable.
To enable attribute routing, add the method in the method of your class: Program.cs
app.UseAuthorization();
app.MapControllers(); // Enables attribute routing
app.Run();
3. Implementing Attribute Routing
To apply attribute routing, you annotate your controllers and actions with the attribute. Here’s how to define a simple attribute route:[Route]
[Route("api/products")]
public class ProductsController : Controller
{
[HttpGet("")]
public IActionResult GetAllProducts()
{
return Ok("All Products");
}
[HttpGet("{id}")]
public IActionResult GetProductById(int id)
{
return Ok($"Product {id}");
}
}
4. Using Route Constraints
Route constraints let you restrict how the parameters in the URL are matched. They are useful for ensuring that a route is only selected if the parameters meet certain conditions. Here’s an example of using route constraints:
[Route("api/products/{id:int}")]
public IActionResult GetProductById(int id)
{
return Ok($"Product {id}");
}
This route will only match if the is an integer.id
5. Combining Routes
You can combine routes at both the controller and action levels to design more complex routing structures. Here’s how to combine routes:
[Route("api")]
public class ProductsController : Controller
{
[Route("products")]
[Route("items")]
public IActionResult GetAllProducts()
{
return Ok("All Products or Items");
}
}
This controller will respond to both and URLs. /api/products/api/items
6. Route Naming and Link Generation
Naming your routes can simplify the generation of URLs in your application. You can refer to these routes by name when generating URLs programmatically:
[Route("api/products/{id:int}", Name = "GetProductById")]
public IActionResult GetProductById(int id)
{
var url = Url.Link("GetProductById", new { id = 123 });
return Ok($"Product URL: {url}");
}
Attribute routing in ASP.NET Core MVC allows for precise control over application routes, making your application easier to manage and extend. By using the techniques discussed, you can organize your routing logic in a way that enhances the maintainability and readability of your code. Remember to experiment with different routing configurations to find what best suits your application's needs.