Logging in .NET Core
Updated on
May 24, 2024
Learn to configure and use logging in .NET Core applications, including log levels, providers, and structured logging.
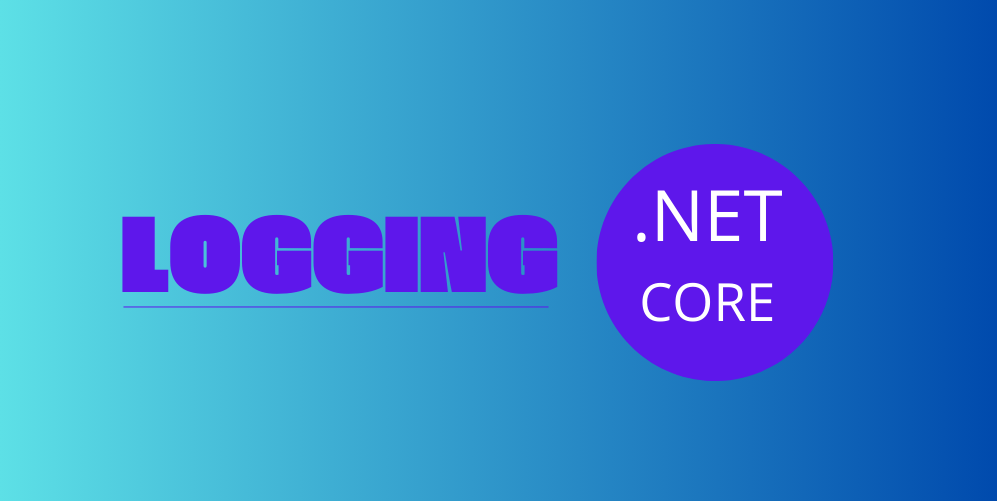
In web application development, logging is an essential part of monitoring, debugging, and maintaining software. Effective logging helps identify problems, understand application behavior, and provide valuable insights into its performance. In .NET Core, logging is a powerful and flexible component that can be configured to meet the needs of any application.
In this guide, we will explore logging in .NET Core in detail. You will learn how to configure logging in your application, use different log providers, and customize logging behavior to suit your specific needs.
What is Logging?
Logging is the process of recording information about the execution of a program. This information can include error messages, warnings, debugging information, and other important data that helps understand what is happening inside the application. Logs are useful for:
- Debugging: Helping find and fix errors.
- Monitoring: Allowing you to track the health and performance of the application.
- Auditing: Keeping a history of operations and transactions performed.
Configuring Logging in .NET Core
Let's start by configuring logging in a .NET Core application. For illustration, we will use a basic project that utilizes ASP.NET Core.
Basic Project Structure
Suppose we have a Program.cs file with the following content:
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddControllersWithViews();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (!app.Environment.IsDevelopment())
{
app.UseExceptionHandler("/Home/Error");
// The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts.
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
app.Run();
Adding Logging
In .NET Core, logging is configured automatically when creating a WebApplicationBuilder. By default, it uses the Microsoft.Extensions.Logging library, which provides support for various log providers such as Console, Debug, EventSource, and others.
To start using logging, we don't need to do much since it's already configured by default. However, we can customize the logging behavior as needed.
Configuring the Logger in Program.cs
Let's configure a simple logger that logs messages to the console. To do this, we modify the Program.cs as follows:
var builder = WebApplication.CreateBuilder(args);
// Configure logging
builder.Logging.ClearProviders();
builder.Logging.AddConsole();
// Add services to the container.
builder.Services.AddControllersWithViews();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (!app.Environment.IsDevelopment())
{
app.UseExceptionHandler("/Home/Error");
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
app.Run();
In this example, we use the ClearProviders method to clear the default log providers and add the console provider using the AddConsole method.
Using the Logger in Controllers
Now that we have configured the logger, we can use it in our controllers. Here is an example of how to use the logger in a controller:
using Microsoft.AspNetCore.Mvc;
using Microsoft.Extensions.Logging;
public class HomeController : Controller
{
private readonly ILogger<HomeController> _logger;
public HomeController(ILogger<HomeController> logger)
{
_logger = logger;
}
public IActionResult Index()
{
_logger.LogInformation("Accessed the Index action.");
return View();
}
public IActionResult Privacy()
{
_logger.LogInformation("Accessed the Privacy action.");
return View();
}
[ResponseCache(Duration = 0, Location = ResponseCacheLocation.None, NoStore = true)]
public IActionResult Error()
{
_logger.LogError("An error occurred in the application.");
return View(new ErrorViewModel { RequestId = Activity.Current?.Id ?? HttpContext.TraceIdentifier });
}
}
In this example, we inject an instance of ILogger<HomeController> into the HomeController. We then use the LogInformation and LogError methods to log messages.
Configuring Log Providers
.NET Core supports several log providers, including:
- Console: Writes logs to the console.
- Debug: Writes logs to the debugger output window.
- EventSource: Writes logs to the EventSource.
To add these providers, you can modify the logging configuration in Program.cs:
var builder = WebApplication.CreateBuilder(args);
// Configure logging
builder.Logging.ClearProviders();
builder.Logging.AddConsole();
builder.Logging.AddDebug();
builder.Logging.AddEventSourceLogger();
// Add services to the container.
builder.Services.AddControllersWithViews();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (!app.Environment.IsDevelopment())
{
app.UseExceptionHandler("/Home/Error");
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
app.Run();
Log Levels
.NET Core supports various log levels, indicating the severity of log messages. The available log levels are:
- Trace: Very detailed log messages. Used primarily for diagnostics.
- Debug: Detailed information used for debugging.
- Information: General information about the application's operation.
- Warning: Indications of potential problems.
- Error: Errors that prevent parts of the application from functioning.
- Critical: Critical errors that require immediate attention.
You can configure the log level in the appsettings.json file:
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft": "Warning",
"Microsoft.Hosting.Lifetime": "Information"
}
}
}
In this example, we configure the default log level as Information and the log level for the Microsoft category as Warning.
Log Filtering
You can filter logs to include or exclude log messages from specific categories or levels. Filtering can be configured in the appsettings.json file or programmatically in Program.cs.
Here is an example of log filtering in appsettings.json:
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft": "Warning",
"Microsoft.Hosting.Lifetime": "Information",
"MyAppNamespace": "Debug"
}
}
}
In this example, we configure the log level for MyAppNamespace as Debug.
Using Third-Party Providers
In addition to built-in log providers, you can use third-party log providers such as Serilog, NLog, and Log4Net. These providers offer advanced features like structured logging, custom sinks, and support for various logging platforms.
Configuring Serilog
To use Serilog, you need to install the Serilog.AspNetCore package:
dotnet add package Serilog.AspNetCore
Next, configure Serilog in Program.cs:
using Serilog;
var builder = WebApplication.CreateBuilder(args);
// Configure Serilog
Log.Logger = new LoggerConfiguration()
.WriteTo.Console()
.CreateLogger();
builder.Host.UseSerilog();
// Add services to the container.
builder.Services.AddControllersWithViews();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (!app.Environment.IsDevelopment())
{
app.UseExceptionHandler("/Home/Error");
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
app.Run();
In this example, we configure Serilog to write logs to the console and replace the default logger with Serilog using builder.Host.UseSerilog().
Structured Logging
Structured logging allows you to log data in a key-value format, making it easier to analyze and query logs. Log providers like Serilog support structured logging.
Here is an example of how to use structured logging with Serilog:
using Microsoft.AspNetCore.Mvc;
using Microsoft.Extensions.Logging;
public class HomeController : Controller
{
private readonly ILogger<HomeController> _logger;
public HomeController(ILogger<HomeController> logger)
{
_logger = logger;
}
public IActionResult Index()
{
_logger.LogInformation("Accessed the Index action at {Date}", DateTime.UtcNow);
return View();
}
public IActionResult Privacy()
{
_logger.LogInformation("Accessed the Privacy action at {Date}", DateTime.UtcNow);
return View();
}
[ResponseCache(Duration = 0, Location = ResponseCacheLocation.None, NoStore = true)]
public IActionResult Error()
{
_logger.LogError("An error occurred in the application at {Date}", DateTime.UtcNow);
return View(new ErrorViewModel { RequestId = Activity.Current?.Id ?? HttpContext.TraceIdentifier });
}
}
In this example, we use format parameters to log the date and time when each action was accessed.
Logging is an essential part of developing robust and scalable applications. In .NET Core, the logging system is flexible and powerful, allowing you to configure and use logs according to your application's needs. With the knowledge gained in this guide, you are prepared to configure logging in your .NET Core applications, use built-in and third-party log providers, and leverage structured logging to gain valuable insights into your software's operation.
No matter the size or complexity of your application, a well-configured logging system is crucial for ensuring its reliability and ease of maintenance. Experiment with different options and find the configuration that best meets your needs.