Configuring SSL in ASP.NET Core MVC Projects
Updated on
May 12, 2024
Learn how to set up SSL to enhance security in your ASP.NET Core MVC projects.
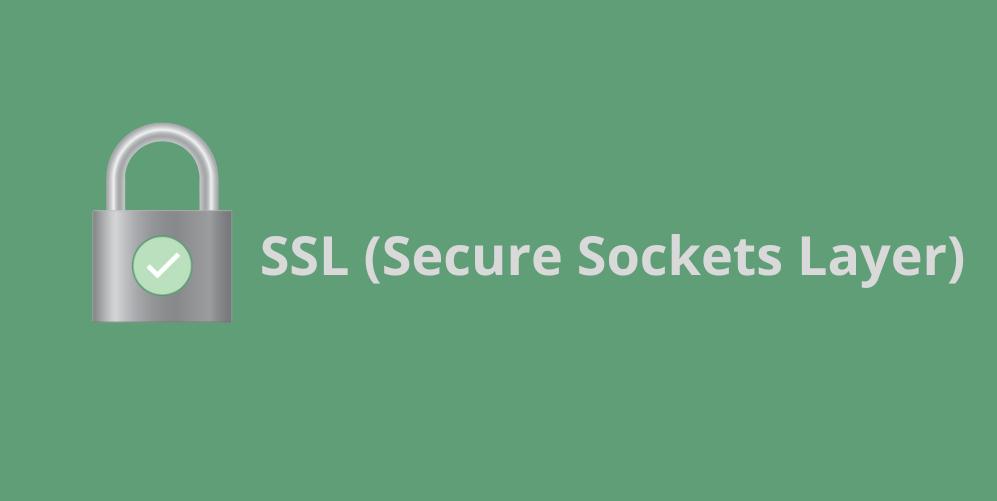
Setting up SSL (Secure Sockets Layer) is crucial for ensuring that communication between the user's browser and the server is encrypted and secure. This tutorial covers how to configure SSL in an ASP.NET Core MVC project.
Prerequisites
- ASP.NET Core MVC installed.
- A valid SSL certificate (can be a self-signed certificate for development).
Step 1: Prepare the SSL Certificate
First, you need an SSL certificate. For a production environment, you should acquire a certificate from a Certificate Authority (CA). For development, you can create a self-signed certificate using PowerShell:
New-SelfSignedCertificate -DnsName "localhost" -CertStoreLocation "cert:\LocalMachine\My"
Step 2: Configure Kestrel to Use SSL
In the Program.cs file, configure the Kestrel server to use the SSL certificate. Add the following code:
var builder = WebApplication.CreateBuilder(args);
builder.WebHost.ConfigureKestrel(serverOptions =>
{
serverOptions.ListenLocalhost(5001, listenOptions =>
{
listenOptions.UseHttps("path_to_certificate.pfx", "certificate_password");
});
});
var app = builder.Build();
// Configure and use the app here
app.Run();
Replace "path_to_certificate.pfx" with the path to your certificate file and "certificate_password" with the password for the certificate.
Step 3: Redirect HTTP to HTTPS
To ensure that all HTTP requests are redirected to HTTPS, add the redirection middleware in the Program.cs file:
var app = builder.Build();
// Enable HTTP to HTTPS redirection
app.UseHttpsRedirection();
// Other configurations and middlewares
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
app.Run();
Step 4: Test the Configuration
After setting up SSL, it is important to test to ensure everything is working correctly. Access your project via https://localhost:5001 and check if the connection is secure, indicated by a padlock next to the URL in the browser.
Configuring SSL in your ASP.NET Core MVC project not only protects information exchanged between the client and server but also enhances user trust in your application. By following these steps, you can easily implement this essential security feature.