How to Use Bootstrap for Responsive Design in ASP.NET Core MVC
Updated on
May 11, 2024
Learn to integrate Bootstrap with ASP.NET Core MVC for responsive, device-friendly web designs.
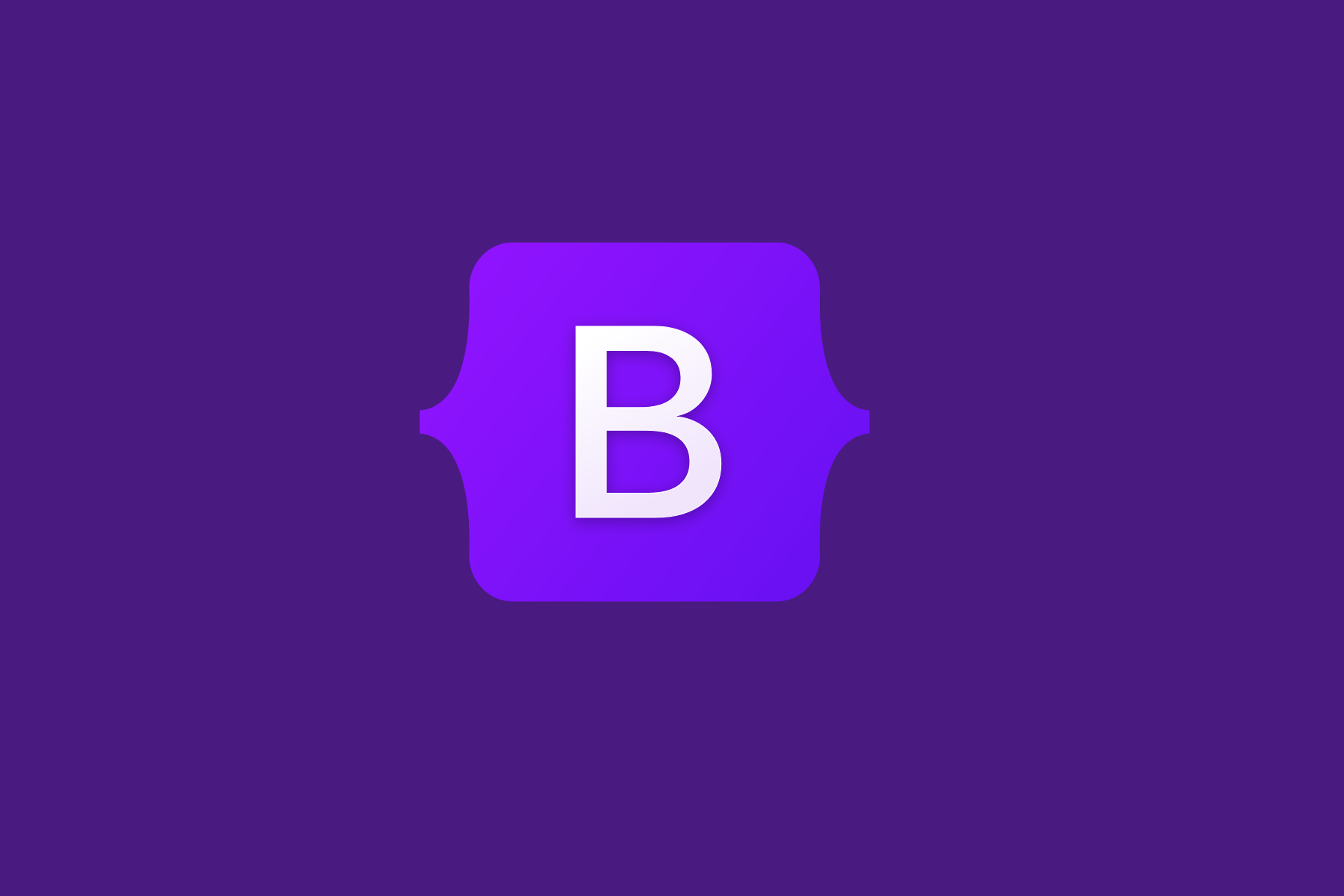
In modern web development, responsiveness is crucial. With the variety of devices used today, from smartphones to desktops, ensuring an interface that adapts perfectly to all screen sizes is essential. By using the Bootstrap framework together with ASP.NET Core MVC, developers can create efficient and visually appealing applications. In this post, we will explore how to integrate Bootstrap into an ASP.NET Core MVC project to develop interfaces that work well on both mobile devices and desktops.
Step 1: Setting Up Bootstrap in ASP.NET Core MVC
To start, you need to add Bootstrap to your ASP.NET Core MVC project. This can be done through NuGet or by including the files directly in the project. The simplest way is via NuGet:
- Open the Package Manager Console (PMC) and run the command: Install-Package bootstrap.
- After installation, make sure the Bootstrap files are referenced in your layout file (_Layout.cshtml), including the links to Bootstrap's CSS and JS files.
Step 2: Using Bootstrap’s Grid System
Bootstrap is renowned for its flexible grid system, which makes it easy to create responsive layouts. The grid consists of containers, rows, and columns that help organize content responsively. Here’s an example of how to use the grid:
<div class="container">
<div class="row">
<div class="col-md-8">Main content</div>
<div class="col-md-4">Sidebar</div>
</div>
</div>
In this example, col-md-8 and col-md-4 are classes that determine how each column will behave on medium-sized devices like tablets.
Step 3: Creating Responsive Navigation
The navigation bar is another crucial component in many web applications. With Bootstrap, you can easily create a responsive navigation bar that adapts to different screen sizes:
<nav class="navbar navbar-expand-lg navbar-light bg-light">
<a class="navbar-brand" href="#">My Application</a>
<button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarNav">
<ul class="navbar-nav">
<li class="nav-item active">
<a class="nav-link" href="#">Home <span class="sr-only">(current)</span></a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Features</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Pricing</a>
</li>
</ul>
</div>
</nav>
Integrating Bootstrap into ASP.NET Core MVC projects is an effective way to create responsive interfaces that offer a great user experience on any device. With these basic steps, you can begin to explore Bootstrap's features more deeply and enhance the interactivity and design of your web applications.
Try implementing Bootstrap in your next ASP.NET Core MVC project and see the difference in responsiveness and design of your application!