Configuring Lowercase URLs in ASP.NET Core MVC for Improved SEO
Updated on
May 11, 2024
Learn how to configure all ASP.NET Core MVC routes to use lowercase URLs for better SEO.
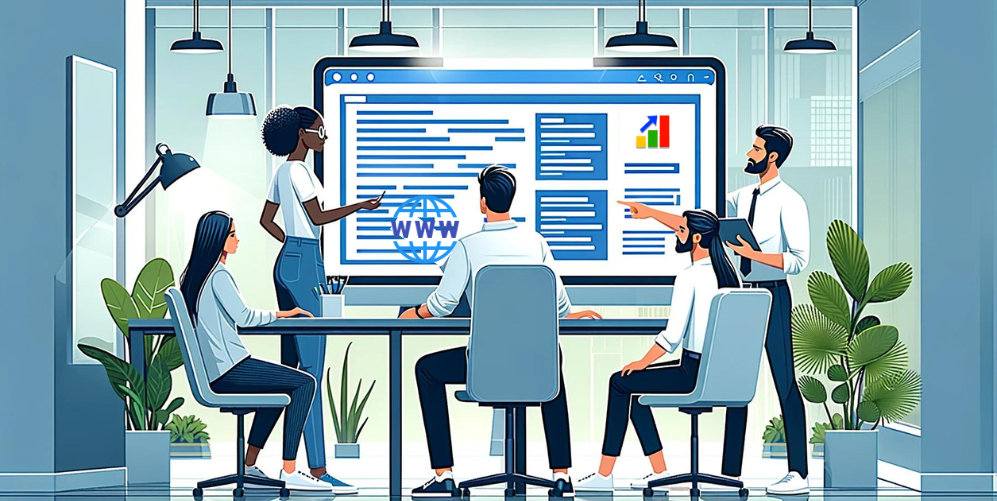
To configure all routes to use lowercase letters in ASP.NET Core MVC, including areas and identity, you can follow the steps below. This setup helps maintain URL consistency and improves your site's SEO.
1. Create the Route Transformer:
Create a new class named that implements. LowerCaseParameterTransformer
public class LowerCaseParameterTransformer : IOutboundParameterTransformer
{
public string TransformOutbound(object value)
{
if (value == null) { return null; }
return value.ToString().ToLowerInvariant();
}
}
2. Add the lowercase routes service:
First, you need to add the lowercase routes service to your project. This can be done by modifying the ConfigureServices method in the Program.cs file.
Add the following code:
builder.Services.Configure(options =>
{
options.ConstraintMap["lowercase"] = typeof(LowerCaseParameterTransformer);
});
This code configures the routing system to automatically generate URLs in lowercase.
3. Configure the routing middleware:
In the Configure method, you need to set up the routing middleware to use lowercase routes. This is done in the Program.cs file, ensuring that routing is added with lowercase URL configuration.
Your code might look like this:
var app = builder.Build();
// Middleware configurations
if (!app.Environment.IsDevelopment())
{
app.UseExceptionHandler("/Home/Error");
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthentication();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllerRoute(
name: "default",
pattern: "{controller:lowercase=Home}/{action:lowercase=Index}/{id?}");
});
app.Run();
After making these configurations, it's important to test your application to ensure that all URLs are being redirected to the lowercase format correctly. Check both standard routes and area routes, if any.
By following these steps, you can configure all the routes in your ASP.NET Core MVC project to use lowercase URLs, including areas and identity, providing better uniformity and SEO optimization for your application.