Enhancing Modularity and Efficiency in ASP.NET Core MVC with Reusable Components
Updated on
May 09, 2024
Learn to build efficient, modular ASP.NET Core MVC apps with reusable components.
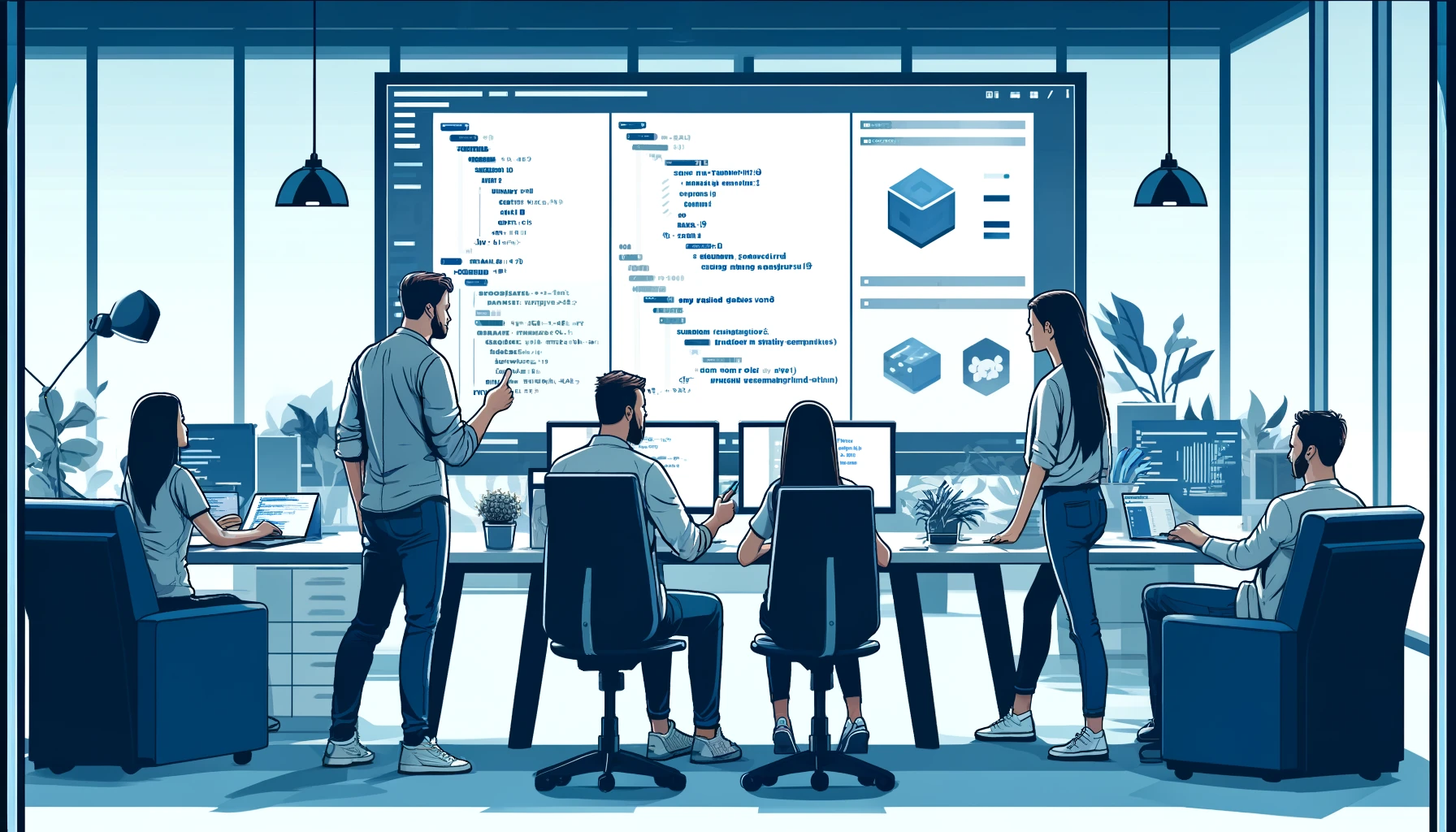
In this post, we explore how to enhance efficiency and modularity in your web development projects using ASP.NET Core MVC. This framework provides several tools to facilitate componentisation and code reuse, such as partial views, view components, and Tag Helpers.
Partial Views
Partial views are an excellent method for breaking down complex views into simpler, manageable sections. They are ideal for isolating parts of a webpage—like a form or a navigation bar—that you want to reuse across various views. To implement a partial view, create a .cshtml file and reference it in your main views using the @Html.Partial or @Html.RenderPartial methods. Here's a basic example of how to implement a partial view:
@Html.Partial("_MyPartialView")
// In your partial view (_MyPartialView.cshtml)
<p>This is a reusable partial view!</p>
View Components
View components are more sophisticated than partial views, integrating both logic and rendering capabilities. They are particularly useful for dynamic content. A view component comprises a class for logic and a view for rendering. To use a view component, you can invoke it within your view using the @Component.InvokeAsync method:
// Invoke a view component in a view
@await Component.InvokeAsync("NameOfViewComponent")
Tag Helpers
Tag Helpers allow server-side code to participate in creating and rendering HTML elements in Razor files. They simplify complex HTML markup, making your code cleaner and more readable. For example, you could create a custom Tag Helper to streamline the creation of form fields used repeatedly across your application:
// Custom Tag Helper for a form input
public class EmailTagHelper : TagHelper
{
public string Address { get; set; }
public override void Process(TagHelperContext context, TagHelperOutput output)
{
output.TagName = "input";
output.Attributes.SetAttribute("type", "email");
output.Attributes.SetAttribute("value", Address);
}
}
By incorporating these techniques into your ASP.NET Core MVC projects, you can achieve more maintainable and scalable applications. Start integrating partial views, view components, and Tag Helpers to see significant improvements in your development process and a reduction in code redundancy.